What does related_name on ForeignKeyField or ManyToManyField in Django do?
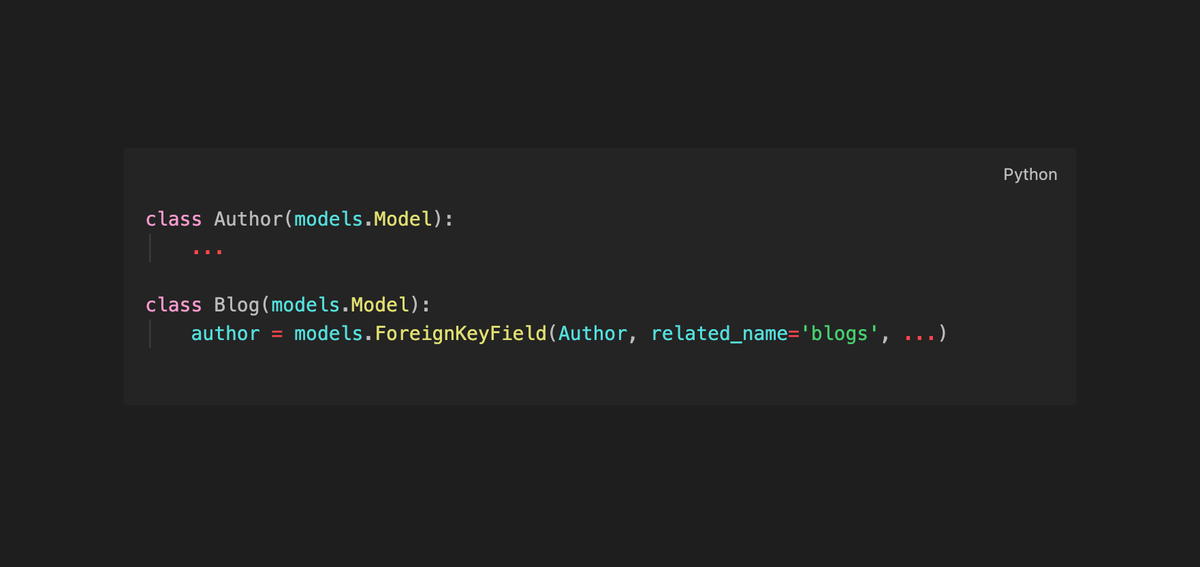
The foreign key (or many-to-many) gives you a way to access/connect another model. related_name
is how you can access the reverse relation: from that other model back to the one you've added the field to.
If related_name
is not set, the default is the model name + _set
. For example: blog_set
. This means if you wanted to get all the blogs for a certain author, you would be using something like this: Author.blog_set.all()
Let's look at an example. I'll keep using a ForeignKeyField example, but it should work the same for ManyToManyFields as well.
How it works
Say you've got a model, Blog
and a model, Author
which are connected on a ForeignKeyField:
class Author(models.Model):
...
class Blog(models.Model):
author = models.ForeignKeyField(Author, related_name='blogs', ...)
Right now, it's easy to see that we can get the Author from a Blog, but when we want to get the Blogs from an Author (reversing the relationship), we'll use the related_name
.
This related_name
allows you to make it easier to understand what you're getting. blog_set
just doesn't communicate as well to me, nor does some kind of complicated class name which makes the default xyz_set convention confusing, like featurebenefitcomparison_set
.
By having the related_name
set, it can be easier to read your code. For example, assuming you have gotten an object and set it to some_author
, we can use our custom related_name
like this: some_author.blogs.all()
. It will grab all the instances of Blog that have some_author
set as the Blog's author.
Choosing the right name
A pattern you can think of for naming the related_name
would be: pluralize the class you're currently add the ForeignKey to. If I'm adding an FK field to the Blog class, then the related_name
can be blogs
.
Keep it simple, readable, and remember it's a helper to get back to where you are from the foreign key / many-to-many relation.