Auto-convert All Images to .webp Format, Instant Load Time Improvement
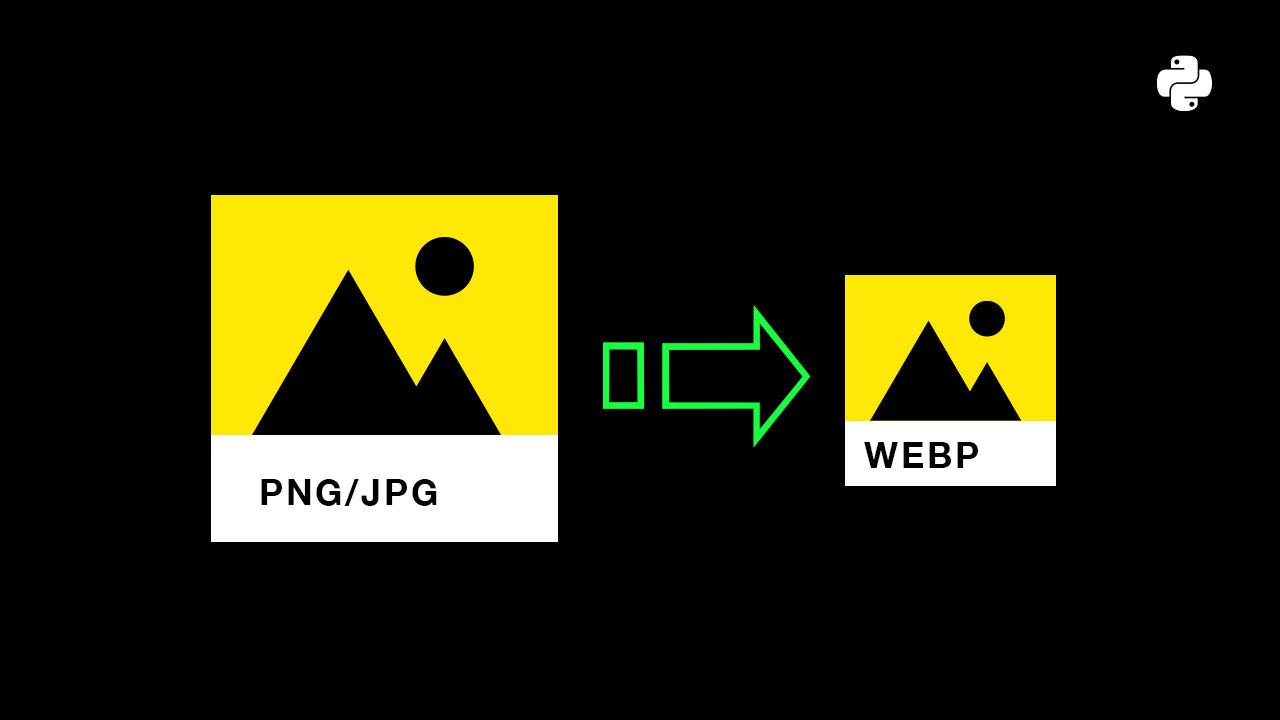
There's no doubt: images hit your site's load time the hardest 9 times out of 10. If you're struggling with performance due to slow load times, audit your images and I would recommend converting them into .webp images. Here's how you can do this with python:
#! python3
# Convert images to webp
from PIL import Image
import os
def convert_to_webp(filename, path="images/"):
extension = filename.split('.')[-1]
fname = filename.split('.')[0]
img = Image.open(path + filename)
if extension == "png":
img.save((path+fname+".webp"), "webp", lossless=True)
elif extension == "jpg" or extension == "jpeg":
img.save((path+fname+".webp"), "webp", quality=85)
def convert_all(path="images/"):
for root, dirs, files in os.walk(path):
for imagefile in files:
if imagefile.endswith(".png") or imagefile.endswith(".jpg") or imagefile.endswith(".jpeg"):
convert_to_webp(imagefile, os.path.join(root, ""))
if __name__ == "__main__":
convert_all()
Note: I made a mistake in my YouTube video! I'm used to putting trailing slashes on the end of paths, so when I pass in the path and when I called this in the video:
convert_to_webp(imagefile, root)
It would throw errors if you had nested folders inside the original path (images, in my case).
We can fix this pretty easily by swapping out line 21 (last line in convert_all function) with this:
convert_to_webp(imagefile, os.path.join(root, ""))
This os.path.join(root, "") fixes the missing trailing slash issue that occurs if we only used root. Passing the empty quotes ("") tells the .join function to simply build the path with no extra directories or paths.
Props to Brahim Rezzoug for asking about how to get nested files to work in this script. Thank you!