Django Models Folder Structure
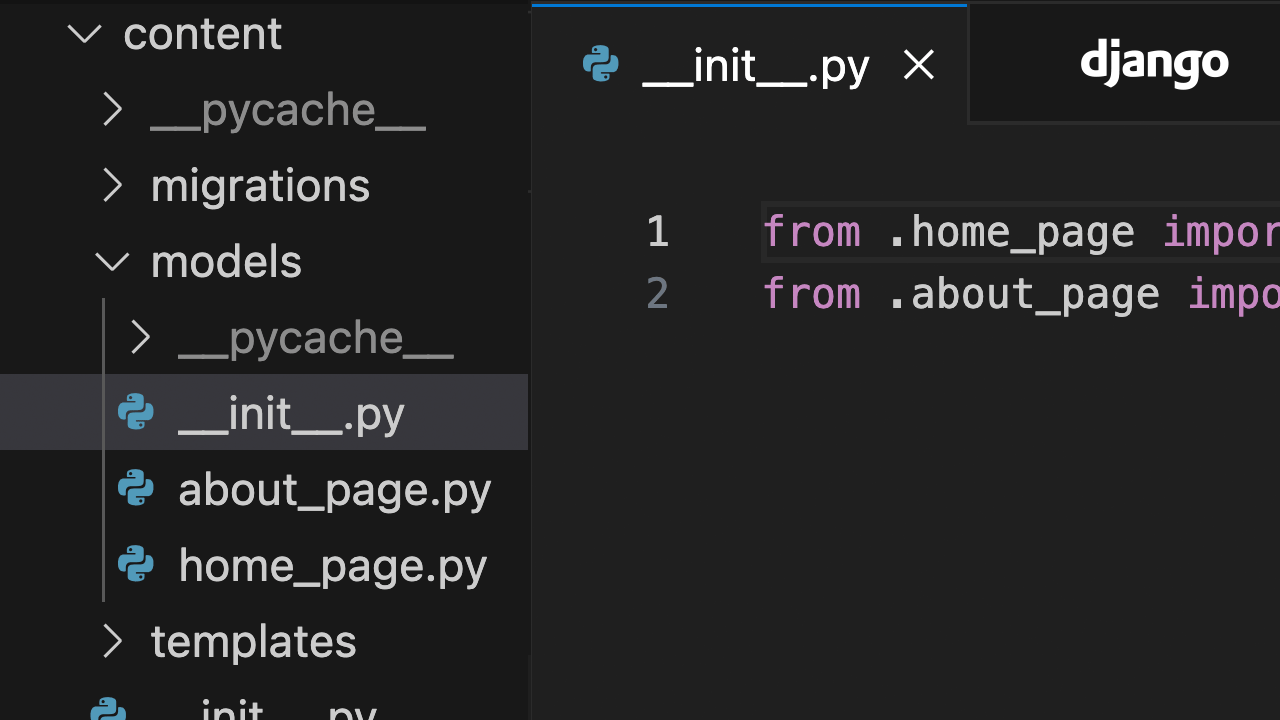
When starting a new app, I recommend immediately creating a models folder and using that to hold files for each model. The advantage in doing this at the start is that your app is easier to maintain as it grows.
It's a lot easier to find the model you're looking for in this structure than it is to look for it in a file that is thousands of lines long with multiple models.
Creating the Directory
Here is how your models structure will look:
my_main_project/
├── my_app/
│ └── models/
│ ├── __init__.py
│ ├── my_first_model.py
│ ├── my_second_model.py
│ └── ...
└── ...
If you're working on a brand new app with no pre-existing models, you can simply set up this folder structure and delete the empty models.py
file that was generated by the startapp
command. You will need one more thing for this to work, so go to the next section below for importing your models.
If you have pre-existing models defined in your models.py
file, you can cut and paste them into their own files inside of the models/
folder. When you've moved out all of the models, you can delete the models.py
file, just make sure you've also imported all of those models (see next section below).
Bundling Your Models Together Through Imports
In order for this folder structure to work, you must have an __init__.py
file inside your models directory. This will hold your import statements that import all of your models from their respective model files.
For example, we can import our models from the two model files into the __init__.py
file like this:
from my_first_model import ModelOne
from my_second_model import ModelTwo, ModelTwoB
Sometimes I'll still end up with two or three models in my model file, but usually that's for inline models or models that are directly related to each other through ForeignKeyFields or ManyToManyFields, which is why the example above has a ModelTwoB
importing from the my_second_model
.
My Process
Create a new app with:
django-admin startapp <app_name>
- Create a folder called
models
within theapp_name
folder - Create a new file inside
models
called__init__.py
- Create a new file inside
models
for the first model and add the model Class definition - Open the
__init__.py
file and import the new model from the file it is found in. - Run
makemigrations
andmigrate
to test that the model(s) have been found successfully
Continue to add new files and import statements as the app grows!
Even if it doesn't grow, I've still found it much easier to handle over the long-term, since it keeps all of my project's apps in a consistent structure and it's easy to navigate through.