Generate n Number of Random Letters with Python
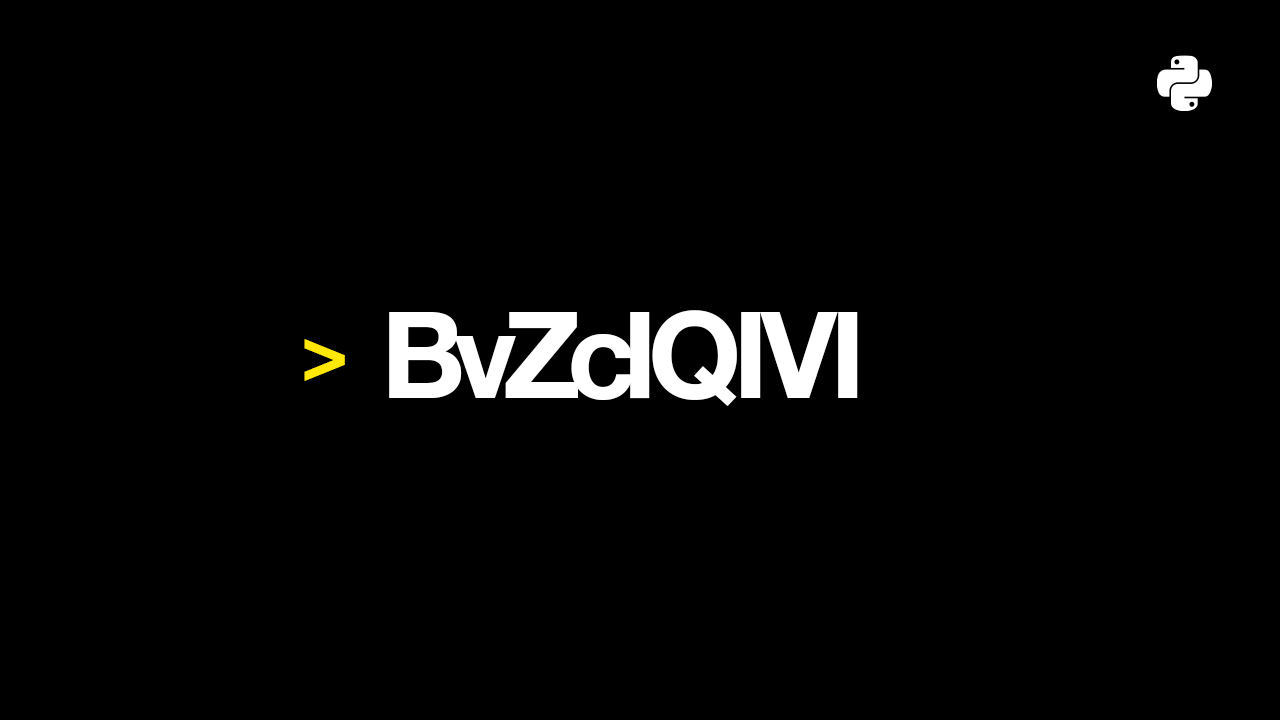
Whether I'm trying to add random letters to a filename or URL to make sure it's unique, this little snippet is nice and fast.
>>> import string, random
>>> random.choices(string.ascii_letters, k=5)
['e', 'I', 'F', 'E', 'Z']
>>> t = random.choices(string.ascii_letters, k=5)
>>> ''.join(t)
'mQprK'
In this function, we can put any number we want, set to the 'k' argument and it will generate that number of random ascii letters:
random.choices(string.ascii_letters, k=23)
Since this returns a list of letters, we use the ''.join... on the list or the function call to pump out our random letters all in one string.
Another way to add to the uniqueness of the string of characters is by tacking on a timestamp at the end. In the snippet below, I've gotten datetime.now() formatted to show the minutes, seconds, and milliseconds all in one string of characters.
>>> import string, random
>>> from datetime import datetime as dt
>>> # put it all together with a datetime string
>>> ''.join(random.choices(string.ascii_letters, k=7)) + dt.now().strftime('%M%S%f')
'EEWTkUT2159430430'
The "random" library/module is a really useful one to keep in your python tool belt, since it could be used in projects like a script that chooses a random winner from a list of names, or perhaps in games or other interactions that need a component of chance.
It is important to note, however, that it is not recommended to use this module for security applications like generating tokens / passwords.
For more randomness, here's a quick guide to getting random numbers, letters, tokens (see the secrets library in action), and choices from a list: