Get the Extension of a Filename in Python
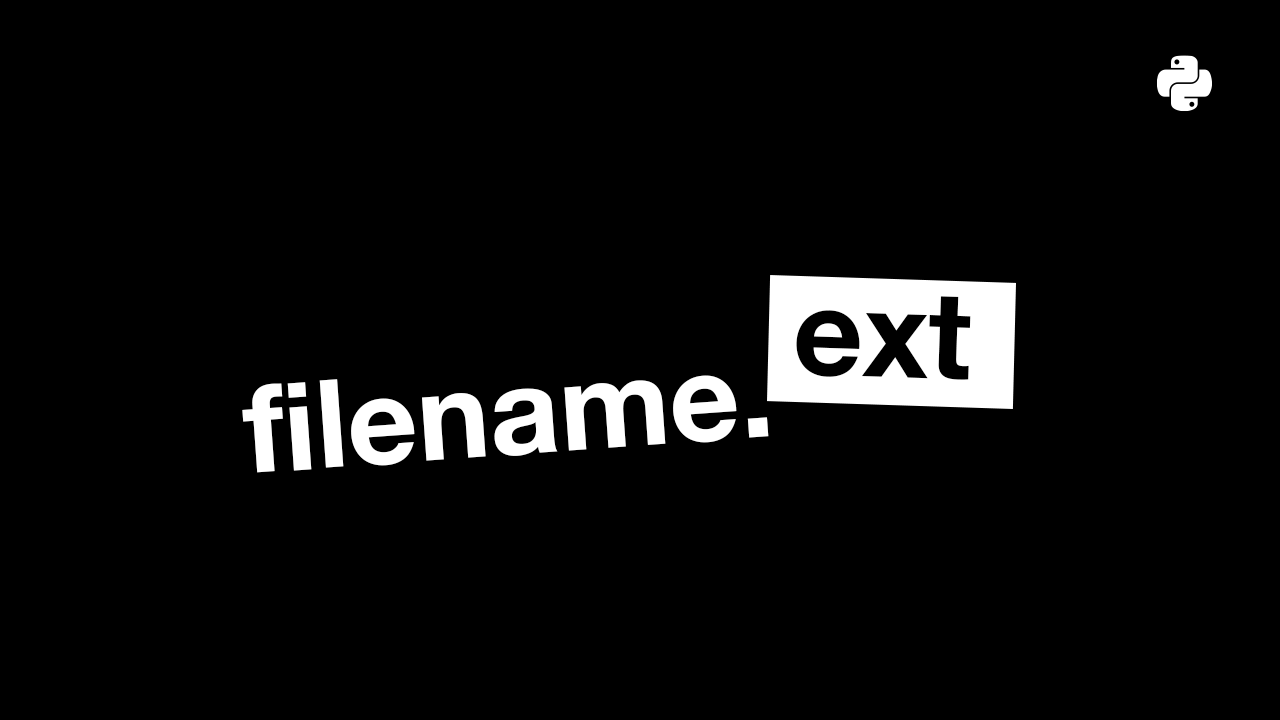
Here's a great little code snippet that can teach you a couple of concepts in python:
filename = "test.html"
extension = filename.split('.')[-1]
The breakdown
We've started with a filename. This can be anything, even a filename with multiple periods in it.
We want to get the extension, but we don't know how many characters it could be. Yes, in the example above, "html" is 4 characters, but what if your filename gets passed in by user input in the future? Maybe you're handling multiple kinds of files: .pdf, .html, .js.
By taking the filename and splitting it on the "." we're able to take the last group after a period and it should be the extension. To illustrate, here's a filename with multiple periods:
>>> filename = "filename.extra.doc"
>>> groups = filename.split('.')
>>> print(groups)
We print the groups to see what happened with the .split('.') command:
['filename', 'extra', 'doc']
This is why if we then get the last item in the list, we'll be getting the file extension:
>>> groups[-1]
'doc'
This approach is much more simple than trying to guess your expected file types, plus there's nothing hard-coded, so it's easily placed in multiple projects.