How to Fix Django Error: Related Field got invalid lookup: icontains
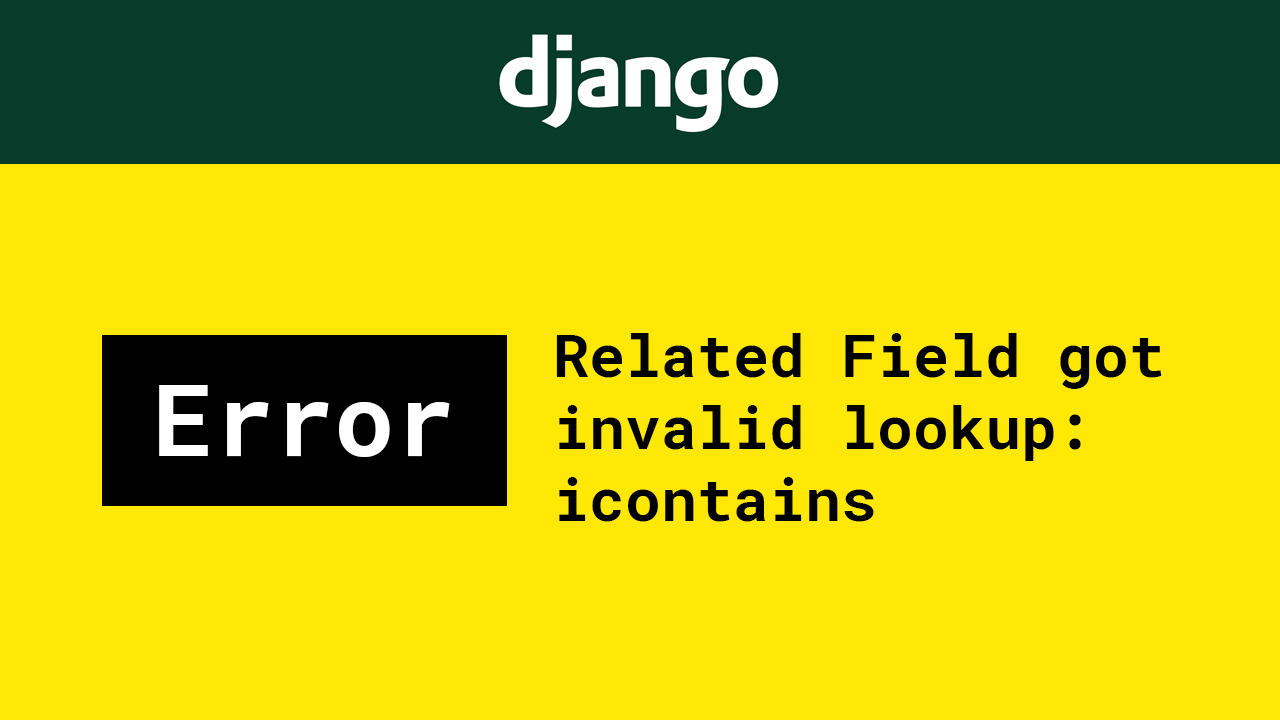
This error typically relates to trying to add a field to your search_fields, but the field is not actually text—perhaps it’s a ManyToManyField or a ForeignKey.
Given the following, we want to be able to search our tags when we're in the Blog List View in the admin:
class Blog(models.Model):
tags = models.ManyToManyField('blog.Tag', blank=True)
...
class Tag(models.Model):
name = models.CharField(max_length=50)
...
...and right now your admin.py's search_fields looks like this:
class BlogAdmin(ApprovalAdmin):
...
search_fields = (..., 'tags',)
...
Since the field 'tags' is a ManyToManyField, we can't do a text search on it, so we need to change that to include a text-based field that belongs to 'tags.' We'll change it to this:
class BlogAdmin(ApprovalAdmin):
...
search_fields = (..., 'tags__name',)
...
Using the double-underscore, we're telling Django: grab the name
field from the tags
that were chosen on Blog instances and search them.
This should hopefully fix your error!