How to Get the First X or Last X Items in a Python List
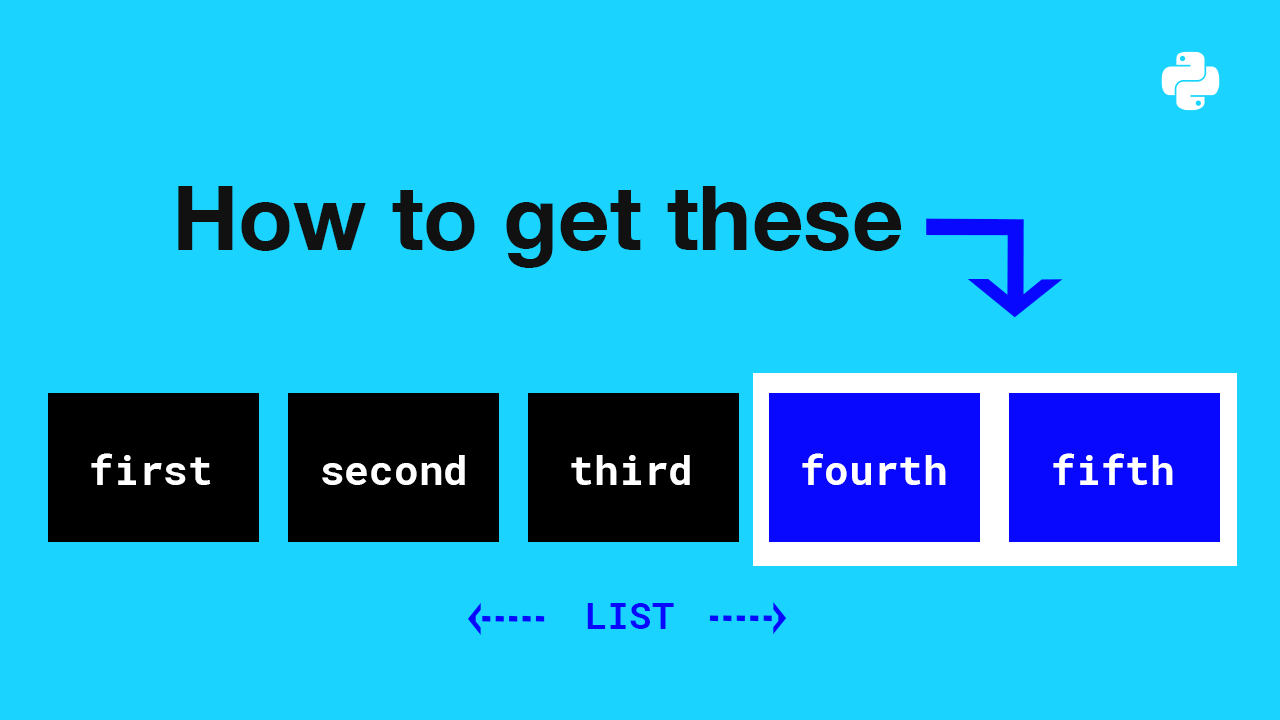
We can use the colon (:) to get the first n (number) of items in a list, the last n items, and some combo of those in between. This is called "slicing" in python. Say we have a list of four items:
>>> my_list = ['a','b','c','d']
First and Last Items
Here's how we get the first two items of the list:
>>> my_list[:2]
['a', 'b']
Get the last two items like this:
>>> my_list[2:]
['c', 'd']
In these cases, you can think of the colon (:) as a marker of one of the ends of the list (the start or the end). You'll place the number of items you want in the square brackets, and put the colon in front of the number if you want the items at the beginning of the list. Putting the colon after the number means you want that many items from the end of the list.
Ranges
You can also use the colon as though it were a range selector:
>>> my_list[2:3]
['c']
>>> my_list[1:3]
['b', 'c']
Remember that indexes start with zero (0). The other tricky thing to remember with this range is that the last number is not included in the returned list. This is why the first example (my_list[2:3]) only returns ['c'], and not ['c', 'd'].
If you want to go more in-depth, I'd recommend the answers on Understanding Slicing on StackOverflow.