How to Get the Value of a Specific URL Parameter with Regex
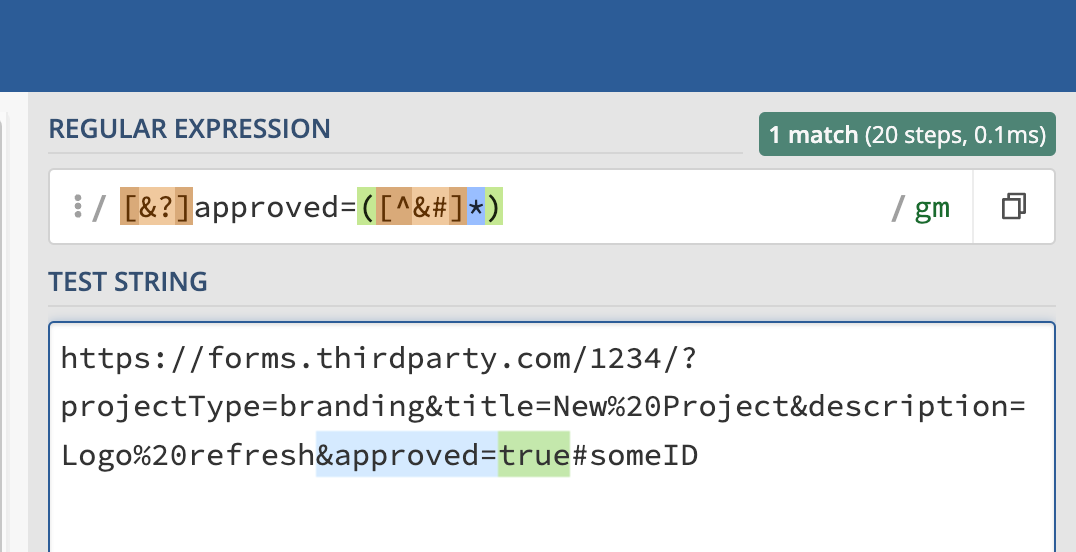
Let's set the scene: you are integrating a third-party form into your page or UI. The third-party integration isn't the most robust, but it does allow you to auto-fill parts of the form using URL parameters.
So if you had a script that could change a parameter value for some present condition, you'll need to find that specific parameter's value. We can do this with regular expressions!
Here's the practice URL:
https://forms.thirdparty.com/1234/?projectType=branding&title=New%20Project&description=Logo%20refresh&approved=true#someID
We have four "fields" to work with:
- projectType
- title
- description
- approved
Building the Regex
We'll target the start of the URL parameters, which always starts with a ?
or if it's not the first param, it'll have a &
in front. So we'll put a single-character set to match these two characters at the start:
[&?]
Then, you can choose one of the field names to target. Let's do the title
for this one:
[&?]title=
The value of a param starts after the =
sign. Now we need to be able to capture any URL-safe characters. This could get tricky, so instead of trying to predict what characters might be present, we can instead look for the end of the param's value.
We know there could be a &
after the value if there continues to be a chain of parameters. We'll start a capture group with ()
and inside of it, we'll look for anything that is not a &
character—using the *
quantifier to greedily grab the other characters:
[&?]title=([^&]*)
This allows us to pull out the value of the title
param:
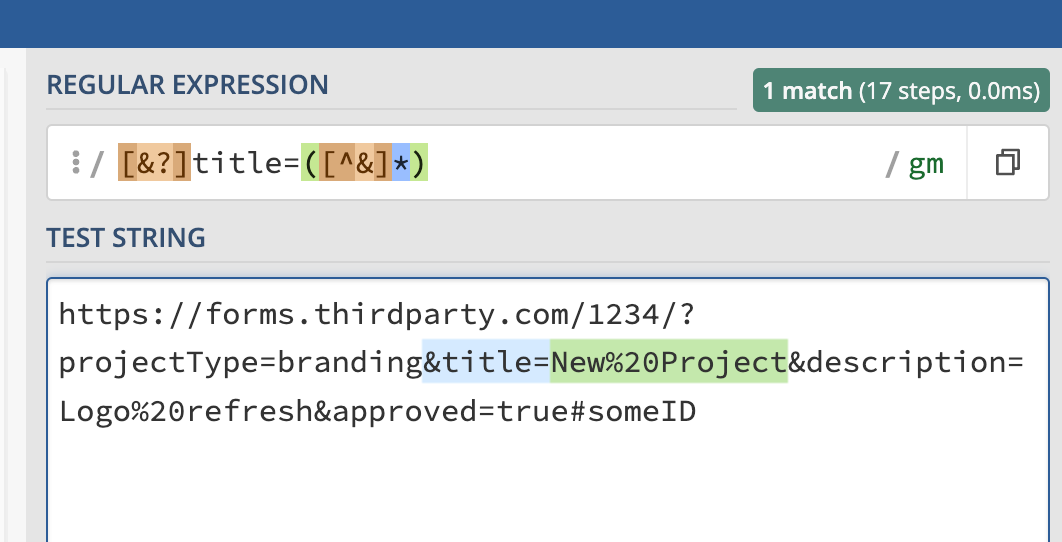
Cleaning up
We're basically there! You should be able to swap out any of the field names (parameters) with title
in our regex and target that specific value.
There's one little hang up that is an edge case, but it's worth the exercise in case there are others that you run into.
Try capturing the approved
field, which is the last parameter in the provided URL:
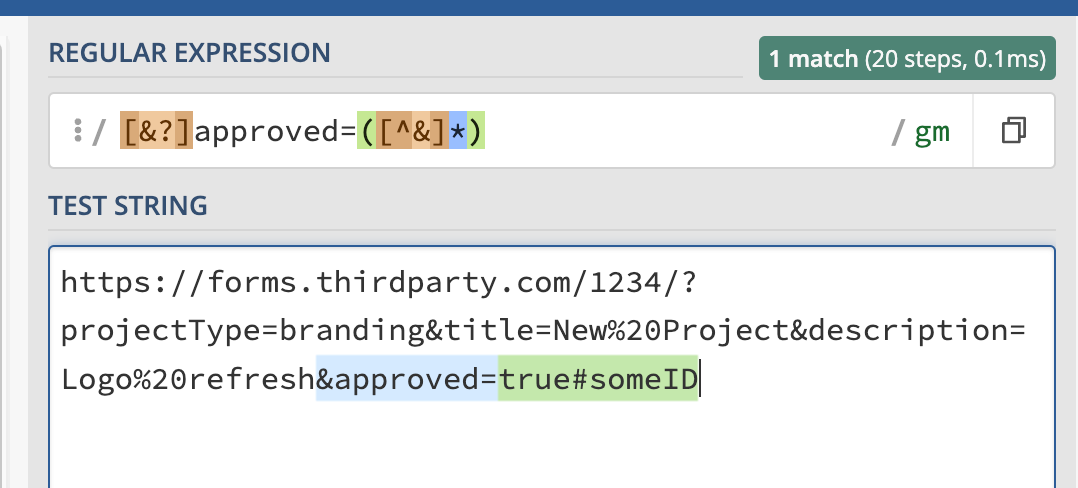
You may notice we captured an ID in addition to the value of the parameter. This could happen by mistake or if someone somehow clicked an in-page anchor link and the ID got tacked to the end of the URL.
Here's the quick fix: adding the #
character to our exclusion set:
[&?]approved=([^&#]*)
Now we're ignoring that CSS ID and only capturing the value:
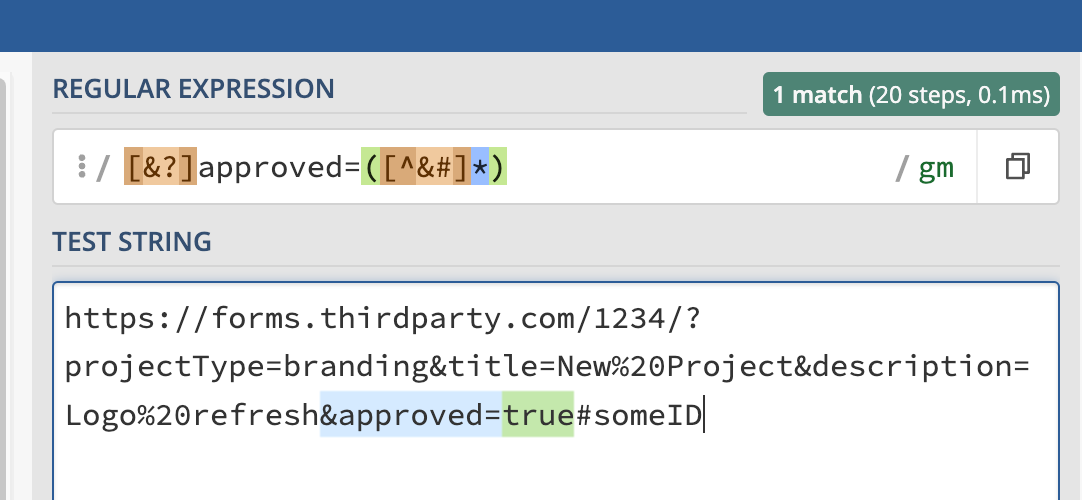