One Lucky Winner! (Choose Someone at Random with a Python Script)
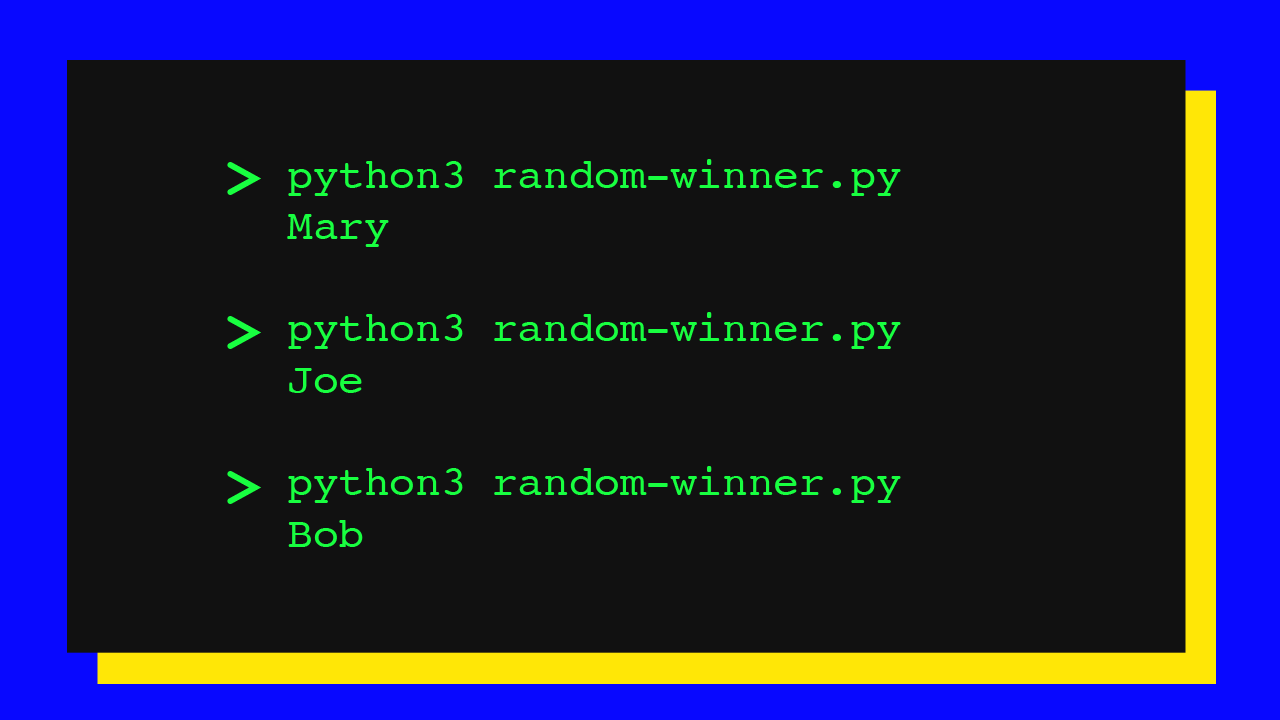
Every marketer or team-lead needs one of these handy. You've got a list of names for a contest or a drawing and you need to choose one person at random. However, to save on trees, we're keeping this all digital. Here's how you can drum up some fun in python in about 2 minutes:
This library comes for free (no extra install required) in python 3.5+:
import random
Which means we can utilize the method, random.choice(<list goes here>) to choose our one lucky winner from the list of names.
Here's the full script:
#! python3
# Choose a random winner from a list of names
import random
def chooseName(name_list):
return random.choice(name_list)
if __name__ == "__main__":
name_list = ["Jess", "Joe", "Bob", "Mary", "Kristen", "Sam"]
print(chooseName(name_list))
Running the script a few times gave me these results:
username scripts % python3 random-winner.py
Sam
username scripts % python3 random-winner.py
Sam
username scripts % python3 random-winner.py
Mary
username scripts % python3 random-winner.py
Joe
username scripts % python3 random-winner.py
Bob
username scripts % python3 random-winner.py
Kristen
Beyond the Basics: Getting Data from Files
If we want to make this even better, we can add functions to read in our names from JSON or CSV files.
Assuming a folder structure like this:
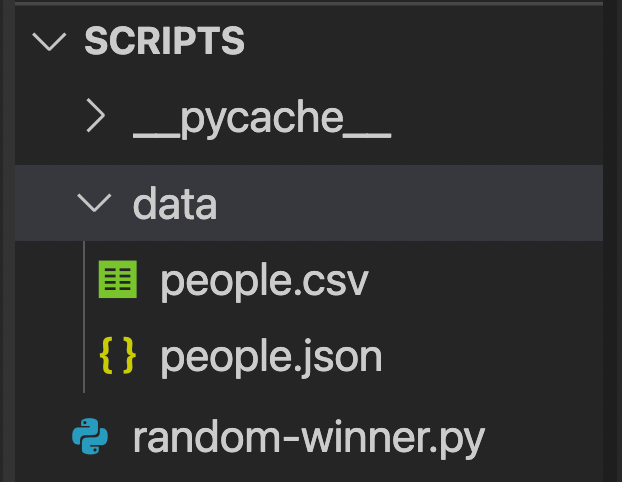
...and CSV or JSON files like this:
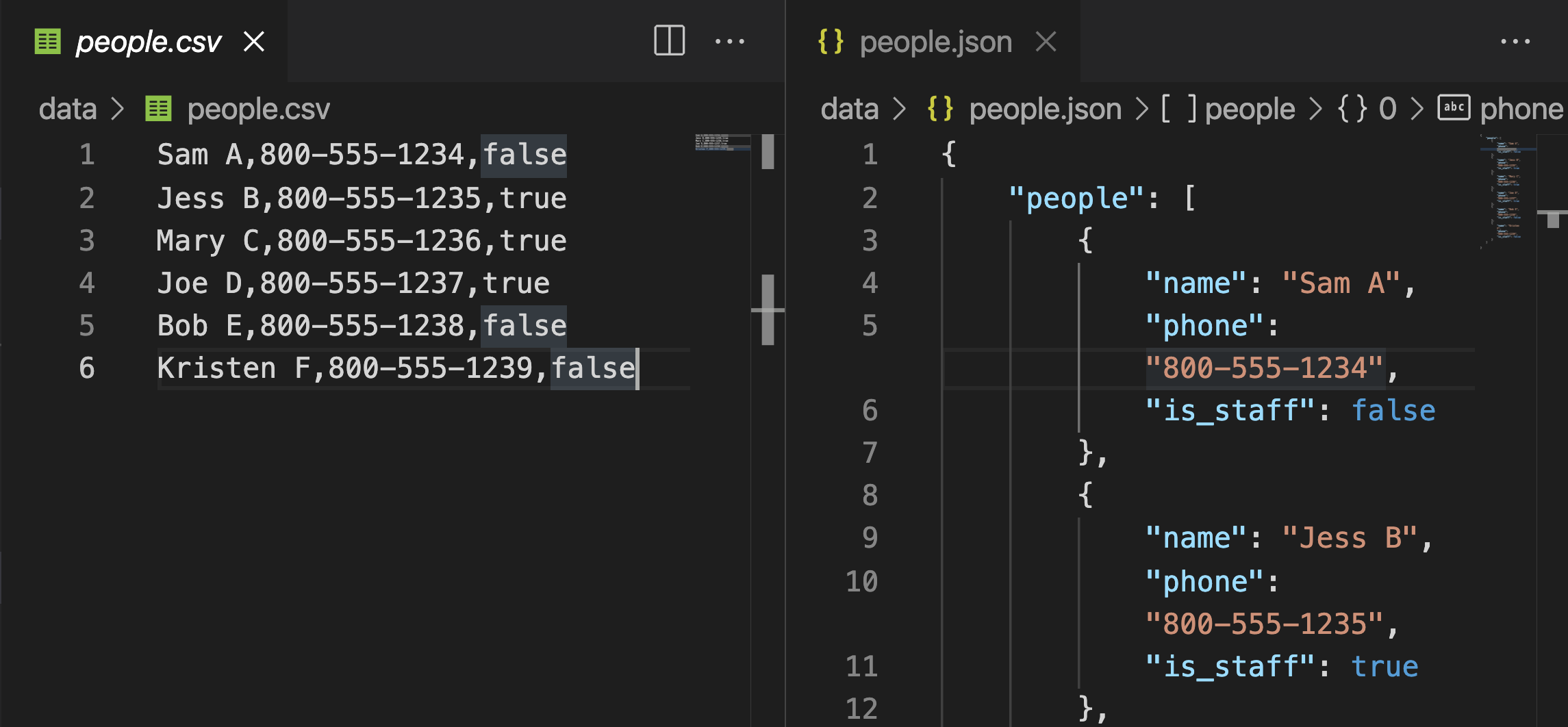
Get Names from CSV File
We can add a function to read in the CSV file and output just the names as a list:
import random, csv
def get_names_from_csv(filepath):
name_list = []
with open(filepath) as data_file:
data = list(csv.reader(data_file))
for row in data:
if row != None:
name_list.append(row[0])
data_file.close()
return name_list
Which could then be used like this to choose a winner from the CSV file:
csv_list = get_names_from_csv('data/people.csv')
print("Lucky CSV winner:", choose_name(csv_list))
Get Names from JSON File
We can also add a function to read in the JSON file and output just the names as a list:
import random, csv json
def get_names_from_json(filepath):
name_list = []
with open(filepath) as data_file:
data = json.load(data_file)
for person in data["people"]:
name_list.append(person["name"])
data_file.close()
return name_list
Which could then be called like this to choose a winner from the JSON file:
json_list = get_names_from_json('data/people.json')
print("Lucky JSON winner:", choose_name(json_list))
Putting It All Together
#! python3
# Choose a random winner from a list of names
import random, json, csv
def get_names_from_csv(filepath):
name_list = []
with open(filepath) as data_file:
data = list(csv.reader(data_file))
for row in data:
if row != None:
name_list.append(row[0])
data_file.close()
return name_list
def get_names_from_json(filepath):
name_list = []
with open(filepath) as data_file:
data = json.load(data_file)
for person in data["people"]:
name_list.append(person["name"])
data_file.close()
return name_list
def choose_name(name_list):
return random.choice(name_list)
if __name__ == "__main__":
csv_list = get_names_from_csv('data/people.csv')
print("Lucky CSV winner:", choose_name(csv_list))
json_list = get_names_from_json('data/people.json')
print("Lucky JSON winner:", choose_name(json_list))