Pseudo Code
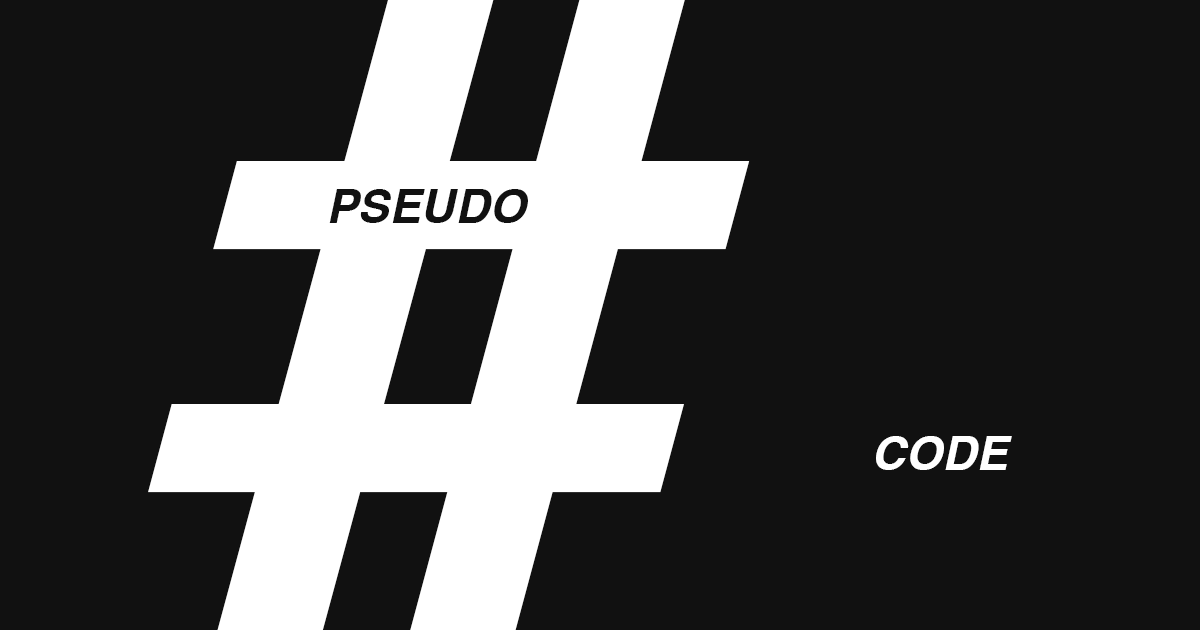
Probably the most boring part of learning to code in the classroom...but in the wild, it is SUPER helpful for starting your project and also when you come back to it a week later.
What is it? Why do I care?
Pseudo code is fake code. It's basically just a list of instructions or steps—the plan for your automation / script. It keeps you motivated, organized, and also helps you understand what's going on in your code.
Writing pseudo code (my way), means we write out what we want the program to do with each step or main idea on its own comment line. Coding languages have ways to add "comments" to the file you're working on, which are basically ignored by the computer when it runs your program.
My go-to language for automation is python, so when we put a "#" at the beginning of a line, it marks the line as a comment and we can type out our steps after it (see example below).
If you've never coded anything before, it's intimidating to just look at code. Having the pseudo code there is like having an outline that you can follow and build on as you learn.
If you have coded before, you know how hard it is to understand someone else's code—and even yours after a little bit. Pseudo code in the comments throughout your script will help you know what's supposed to be happening.
Here's an example of a task I want to automate, stubbed out in pseudo code:
# Get a list of URLs to check for redirects # For each url in the list, # Get the response # Print the history (redirects)
Now that I have this outline, I can fill in the code for each step under each comment. It's a checklist and an explanation at the same time.
import requests # Get a list of URLs to check for redirects urls = ['https://notdefined.tech', 'https://www.revamp-design.com'] # For each url in the list, for url in urls: # Get the response response = requests.get(url) # Print the history (redirects) if response.history: for redirect in response.history: print(redirect.url)
Give it a try! Take one of those tasks you've been wanting to automate and think through the steps you might have to take to get it done. Regardless if you know how to solve it with code, the exercise can reveal inefficiencies even when you're doing something manually.
Having a few pseudo-code descriptions at the ready will help inspire you to learn more about automation and it will help structure what you need to do when you do have enough familiarity to start putting a little code together.