What Case is THAT?
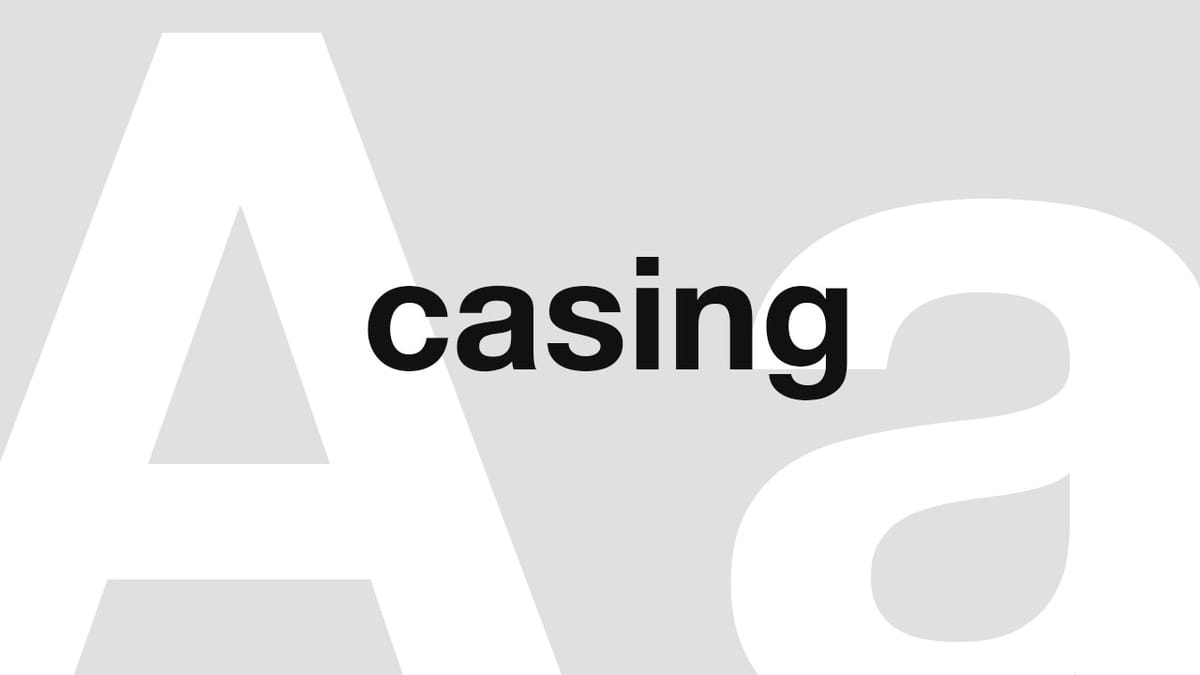
In English and other languages based on an alphabet, casing is used to describe the style of text for when upper and lowercase letters should be used. Sometimes these styles are easy to identify and use; some are more complex than they appear at first. Here's a breakdown of casing types and how to identify and use them.
Title Case
This is one of the seems-simple-at-first casing styles, because you see it everywhere and it looks like you just capitalize the first letter of every word. However, there are details to this case that don't quite fit the rule.
For example, the article, "the," gets a capital letter if it is the first word in the title-cased text, but not if it's somewhere "in the middle." Same goes for the other stop words—usually they are lowercased unless they are the first word.
There may also be different rules depending on the writing style as well, so if you're trying to figure out the title case version for your work or a school assignment, check the style guide for the writing style the organization adheres to.
If you're trying to code up something to validate or auto-capitalize your words in title case, be sure to include all of the rules for this case, don't just stop at:
your_text.title()
Sentence case.
This your typical writing style in long form content (though some brands use it for headlines). Start with a capital letter and all other words are lowercased unless they're a proper noun or an acronym. Basically, the case only dictates the first letter to be uppercase, it does not change any other casing guidelines.
You can get partially there in python with:
your_text.capitalize()
...but it only capitalizes the first letter, so it's not sufficient to cover all of the rules for sentence case.
UPPERCASE OR ALL-CAPS
Using all capital letters for the entire text. This may work for smaller components, but it becomes very difficult to read when multiple words are uppercased, or when scanning and fast comprehension are critical. Use sparingly and intentionally.
your_text.upper()
lowercase
Finding lowercase headings are especially common in avant-garde art or other high-end type treatments. It's the easiest to read, because of the distinctive "island shapes" that it creates. However, it's not common to use in body text and can actually create a negative perception, because it would break the rules of writing to use it in paragraph form, making you appear lazy or uneducated.
your_text.lower()
camelCase
You'll find this in code all the time. It's a common convention for variable names. The rules to identify and use this are: lowercase the first word, capitalize the first letter of every word after; no spaces.
snake_case
Python code anyone? This case is the convention for variable names in python and may be used in other coding languages. The hallmarks are: all lowercase with underscores between words.
SCREAMING_SNAKE_CASE
What a name! Global variables and constants typically use all uppercase variable names so it's easy distinguishable in the code. Python certainly makes use of this case for those constant variables, and you'll see them in config or settings files, as well as other definitions for unchanging variables.
kebab-case
Clean, easy to read URLs will use this casing. Everything is lowercased and words are separated by hyphens. This makes for readable URLs in that tightly-packed URL bar, and it needs to be lowercased, because most servers (Linux-based) are case-sensitive, so you want to make sure the lowercase convention is enforced to avoid URL collisions, and again, it just looks more clean and readable. That's also why my personal preference for filenames is this kebab-case format.
TRAIN-CASE
I'm not sure what the use case for this one is, but it is visually punny. Think of each word as a train box car (uppercasing creates that blocky island shape I referred to earlier) connected by a hyphen. Similar to the kebab case, just all uppercase.
PascalCase
Another code convention where all words start with a capital letter, no spaces between words. In python, we'll see this case mostly for class names. Other languages may also use it for variables and function names. Supposedly, this could also be called UpperCamelCase, since the first letter treatment is the only difference between Pascal Case and Camel Case.
Other Notations
Hungarian notation: strName
Basically camel case, but starting with the type of the variable as the first "word." Supposed to help indicate the type or the purpose of the variable, which could be helpful in understanding code. I've not personally heard of or used this convention professionally, but it may have some esoteric uses.
Initial Caps
Used in technical writing to help readers identify key concepts or perhaps field names or other terms that need extra emphasis.
For example: To make the change, find the Update Name field and then click Save.
In the example above, "Update Name" and "Save" are in Initial Caps to help draw visual emphasis as we're guiding a user through a process.
CamelCaps
Seemingly found most often in creative contexts or branding, CamelCaps capitalizes one or more letters within a word. Think FedEx, WordPress, YouTube.
This may also be called, "medial capitals."
Extra: Using Python to Validate "Code Cases"
More for fun, you can use the .isidentifier()
method on a string to see if it's considered an identifier in python. Here is the list and the output:
>>> sc = "snake_case"
>>> screamer = "SCREAMING_SNAKE_CASE"
>>> kebab = "kebab-case"
>>> camel = "camelCase"
>>> train = "TRAIN-CASE"
>>> pascal = "PascalCase"
>>> sc.isidentifier()
True
>>> screamer.isidentifier()
True
>>> kebab.isidentifier()
False
>>> camel.isidentifier()
True
>>> train.isidentifier()
False
>>> pascal.isidentifier()
True
snake_case | SCREAMING_SNAKE_CASE | kebab-case | camelCase | TRAIN-CASE | PascalCase | |
---|---|---|---|---|---|---|
IDENTIFIER? | True | True | False | True | False | True |